The week 2 problem set wasn’t hard as this week, where we are told to write a program that mimics voting. This Pset took me days before I complete and submit it. I had to re-watch lecture multiple times, make use of the cs50 approved forum (Reddit, StackOverflow), and google to get some of my questions answered.
So if you are doing this pset, you need to understand how to ask questions, especially if you are a novice like me just starting a journey in programming, else you will be prompt to quit early.
I’ve been able to complete the pset3 and in this post, I will discuss all steps that I used just stay tuned.
What to do
like I said earlier, Plurality is all about simulating election through voting, the program will take a sequence of votes as input and will output the winner of that election.
Since we are not writing these code from scratch like the previous pset’s, we are just going to update the pre_written code and complete each empty function’s.
Make sure you utilize the wget command to download the code on your IDE and watch the walk-through to understand it.
Complete the vote
function.
vote
takes a single argument, astring
calledname
, representing the name of the candidate who was voted for.- If
name
matches one of the names of the candidates in the election, then update that candidate’s vote total to account for the new vote. Thevote
function in this case should returntrue
to indicate a successful ballot. - If
name
does not match the name of any of the candidates in the election, no vote totals should change, and thevote
function should returnfalse
to indicate an invalid ballot. - You may assume that no two candidates will have the same name.
Complete the print_winner
function.
- The function should print out the name of the candidate who received the most votes in the election, and then print a newline.
- It is possible that the election could end in a tie if multiple candidates each have the maximum number of votes. In that case, you should output the names of each of the winning candidates, each on a separate line.
Vote Function
we are to update this function, such that it looks for a candidate that corresponds to the user’s voter’s choice, then if candidate found, we should update their vote total and return true.
Pseudocode for the Vote function
- Iterate through the candidate count
- check if both candidate and voter’s choice are the same,
- if so, update candidate votes and return true.
bool vote(string name)
{
//Loop through the Candidate count
for (int i = 0; i < candidate_count; i++)
{
//check if candidate is similar to what is user's vote
if (strcmp(candidates[i].name, name) == 0)
{
//if similar to what user vote for, then increase the specific candidate vote and return true
candidates[i].votes++;
return true;
}
}
//else if not similar to candidate vote return false.....and print invalid vote
return false;
in the above vote function
- we use for loop to loop through the candidate_count (number of candidates in the election).
- then use strcmp, a function that is defined in string.h header, to compare both the candidate and who the voter’s voted for at index [i]. i.e, the name and candidates[i].name respectively.
- then we update the candidate’s vote count by incrementing candidates[i].votes, thus return true for successful implementation.
Print_winner function
Print winner function is task with printing candidate who has the most vote, we are to update it to print the candidate with the highest votes, even if they are two we should print them like that.
This code seems undoable for me at first, until I get this simple pseudocode logic
Pseudocode
- Create a new variable maximum_vote and set it to 0
- then loop through the candidate_count, checking
- if candidate’s votes is greater than the maximum_vote…then
- set maximum_vote to the new candidate’s votes
- Iterate through the number of candidates again, now check
- if the candidate’s vote is similar to the maximum_vote, if so
- print the name of the candidate at that index.
code here
void print_winner(void)
{
//Create and variable and set it to 0
int maximum_vote = 0;
//iterate over list of candidate
for (int i = 0; i < candidate_count; i++)
{
//check for candidate votes that are greater than maximum_vote and set them to maximum_vote
if (candidates[i].votes > maximum_vote)
{
maximum_vote = candidates[i].votes;
}
}
//iterate over list of candidate
for (int i = 0; i < candidate_count; i++)
{
//check for candidate votes that are equal to maximum vote and print them as you go
if (candidates[i].votes == maximum_vote)
{
printf("%s\n", candidates[i].name);
}
}
return;
}
in the above code
- we first create a new variable maximum_vote and assign it to 0.
- then we check if candidate’s votes at index [i] (candidates[i].votes) is greater than maximum vote (maiximum_vote), if so
- then we store the highest vote at candidates vote to maximum_vote by assigning the maximum vote to the candidate’s vote
- we then loop through the candidate votes again to check if the candidate’s vote at index [i] is similar to the maximum vote, which is true, thus printing the candidate’s at that index[i] as we go.
Cs50 Pset3 Plurality full code here
Screenshot from Cs50 check50
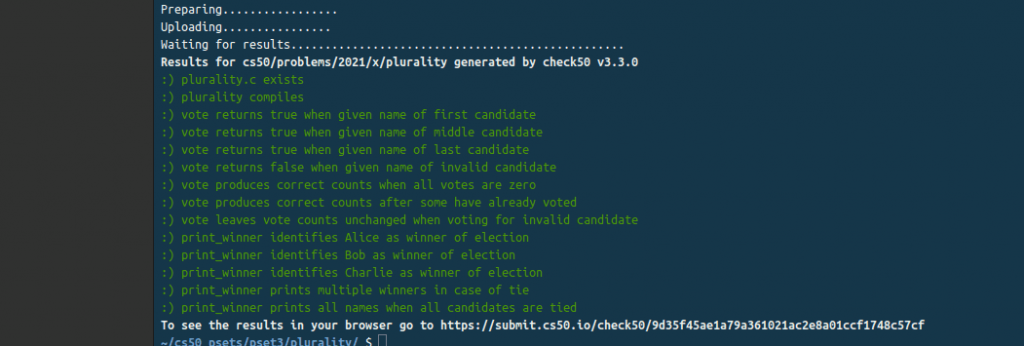
A brief Conclusion
that’s it, I hope you are able to solve and see green after using the above pseudocode and code snippet. you can also check out my summary of the week 3 lecture.
While I’ve been able to post the solution, please don’t just copy the entire code, make sure you understand every line of the code, because this Pset will be like a stepping stone for you in the coming weeks Pset.
So if you are having problem understanding the code or you feel confused, don’t hesitate to use the comment box below, i will reply as soon as possible. Thanks.
Hi
Thanks for the helpful clarification, but whenever I wrote the name of the candidates, it typed Invalid vote.
Hello Aisha, I’m happy you made it to my blog, may I know which specific section is giving you the error (invalid vote), have you tried the above solution?. I got every test cases green with check50 approved with the above solution. Kindly reply to me as I’m happy to know if you’ve got it solved.
Hi,
Still not solved, it kept telling me invalid vote whenever I typed Alice Charlie or Bob!
Maybe, the error is from your input or you are missing something.
I already post a screenshot report from check50 so that you can see that the code is working.
What I will suggest: Copy the above full code and paste it directly on your IDE, then compile with make.
make sure you compile as that might be the probs for your recurring error.
I did what you suggest, it doesn’t work, then I wrote check50, and I got this message:
error: the following arguments are required: slug
THANKS, IT FINALLY WORKS !
I really appreciate your guidance.
it is because you didn’t put return true after the loop