CS101, an online course from Standford University on EDX taught by Nikola Parlante has been the answer to many of my questions and I am so glad I took this course earlier before any other computer science course online.
In this post, I will be writing about what I learnt throughout my journey in the course. Don’t expect to get course content here as this post only contains the overview of what I learn in the course. If you feel like taking this course I will advise you to go enroll on EDX so that you can have more knowledge of the course and also have resources that are not revealed here.
What is CS101 and why did I choose this course?
As written in the Course About page: “CS101 is a self-paced course that teaches the essential ideas of Computer Science for a zero-prior-experience audience. Computers can appear very complicated, but in reality, computers work within just a few, simple patterns. CS101 demystifies and brings those patterns to life, which is useful for anyone using computers today”
I choose this course because I needed to build a foundation in computer science, as i am looking to advance and teach myself programming, I get to know that jumping into programming won’t be the best option but general overview of how computer’s works will help me speed not only my programming journey but also in other things related to computer science.
Cs50 vs Cs101
Oh, I’m sorry….I know you don’t expect to see this, but having check online I found out that most people that are trying to persuade a journey in the Computer science field or Programming often find it hard to distinguish between CS101 and Cs50 as a beginner’s course and which to take among the two.
Having taken the course, my advise is that if you are entirely new to Computer world, then Cs101 will be the best option because you will be introduced to the basics of Computer from the Hardware part, Software and why things work the way they did on it…….on the other hand if you are an experience person that just need to be introduced to programming or want to learn to code, I will strongly advise you to go for Cs50, an Harvard Introduction to computer science and the Art of programming.
My overall take is that, go for Cs101 first and then Pursue Cs50. Cs50 is complex and it’s generally programming while Cs101 is still easy compared to Cs50 and it will help you do more better in Cs50 because some basics required in Cs50 are already taught in Cs101. Either way, both are good beginner’s course.
Let’s Dive in as a explain what I learned in Cs101.
What I learned from CS101 course
The fundamental equation of Computer = Powerful + Stupid
Powerful in the sense that it look through masses of a data, billion of operations can be executed in a seconds and it is Stupid because those operation that it perform are simple and mechanical.
Generally Computer can perform different task as fast as possible, but it can’t make things run by itself. It still needs the knowledge of human beings to tell it what to do and these people are called Programmers.
But how does Computer work?
Computers are driven by code i.e an instruction based language written by programmers so that it can do one or two things. an example of an instruction can be to add two numbers. The steps/procedure by which the programmer used to operate computers is called an AlgorIthm while the language that programmer used to write these instructions is Programming language. In Cs101 the language that is used is JavaScript and the code were not complex.
Code Writing
Code refers to the language that computer can understand. Code is like a lego bricks, individual pieces are super simple until they become great combinations. How the code is being structured is called Syntax. Syntax are set of rules in which code must follow otherwise it will results in error. A Comment is a way one can write notes about a code that is ignored by the computer.
Note: some code written here were not normal part of JavaScript, CS101 added it to make things clear for learner…..and I will be using “#” for explanation in code.
||an example of code
print(6);
print(1,2);
# The "||" is used for commecnt
#print() is a function, just like a verb
Code Variable
Code Variable is just like a box that holds value. Variables work as a shorthand, we assign a value into a variable, and then use that variable on later lines to retrieve that value. In simplest case, variable works to avoid repeating a value: we store the value once, and then can use it many times.
x = 14:
print(x * 2)
#The result here will be 28, We can re-use the "x" variable many times, no need to type x again.
An example is x = 14, the ‘x’ can later be used to multiply or add another number as in x * 2, which will give us 28. The variable ‘ x’ can be used many times after being declared.
Digital Image
Images are everywhere, it looks natural but behind the scene it is made up of tiny numbers. When you zoom in image, you will notice some square pattern in it. these tiny squares are called Pixel and they make up the whole Image. They are quite small, each pixel can represent one color.
An image of 250 pixels wide and 250 pixels high simply has 62,500 pixels. Each pixel stores color information for your image. It will usually store it in 3 components, known as RGB (Red, Green, Blue)
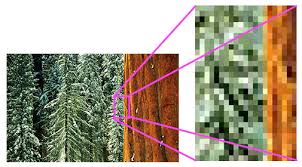
- Each pixel stores color information for your image, for example the picture below shows that Pixel at x, y (1, 0) stores the color “green“……pixel at x y (4, 2) stores yellow and so on.
- Each pixel – small, one color organize pixels as a grid
- Every pixel can be identified by x,y numbers, “address” x=0, y=0 upper left — (0, 0) x=1, y=0 one pixel to the right — (1, 0)
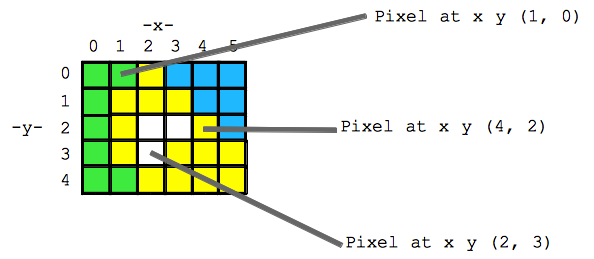
But how do we recognize color of a pixel?
- The red/green/blue (RGB) scheme is one popular way of representing a color in the computer.
- In RGB, a color is defined as a mixture of pure red, green, and blue lights of various strengths. Each of the red, green and blue light levels is encoded as a number in the range 0..255, with 0 meaning zero light and 255 meaning maximum light.
- An example is when we have the RGB (Red, Green, Blue) to be value (255, 255, 0) respectively we have the color “Yellow”
Image Code: in this section of the week 2 lecture we write code to load and manipulate some image by just manipulating one pixel at a time
image = new SimpleImage("x.png");
image.setZoom(20);
pixel = image.getPixel(0, 0);
pixel.setRed(255);
print(image);
- we use the new SimpleImage(“x.png”) to load the image into memory and store it in variable “image”
- then zoom the image by 20 with………..image.setZoom(20);
- then we get the exact pixel to be manipulated with……. image.getPixel(0, 0) and set it the location to Red with pixel.setRed(255)
- then print the image
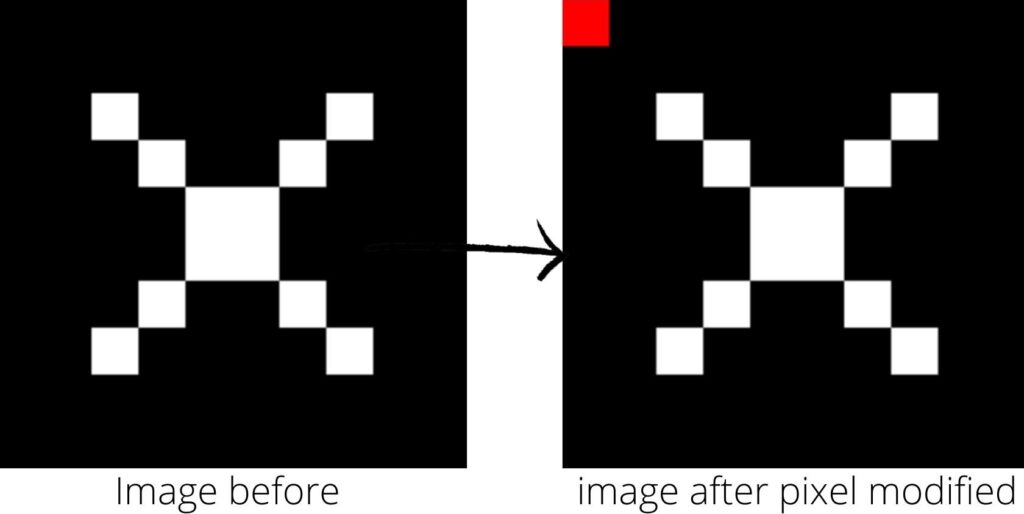
Image for loop
in this lecture, we leaned the for-loop and its use case with image. The “For” Loop is used to repeat a specific block of code a known number of times.
For example: instead of going through all the individual (x,y) coordinates of an image with 457 * 360 pixels — 164,520, a for loop will work fine by looping through and manipulating each point according to our choice.
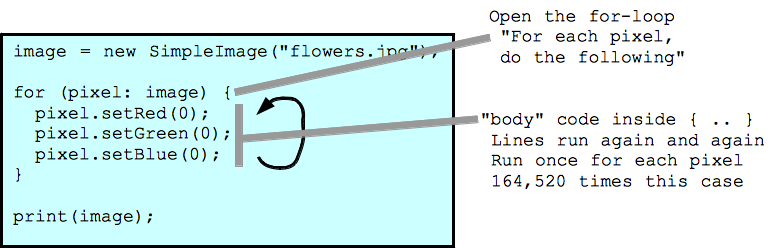
Image Expression
Image Expression: An expression in programming is like performing calculations. e.g x = 5, y = 10….. z = x * y. in this case z is now 50.
in the context of using an expression in a image we can modify any pixel of choice by adding or multiply any digit e.g pixel.setRed(pixel.getRed() * 20)
pixel.setRed(pixel.getRed() * 20)
GrayScale Image:
A grayscale is a black and white form of an image. to get the grayscale of an image, we will need to compute the average value of the RGB and set the individual RGB value to the average.
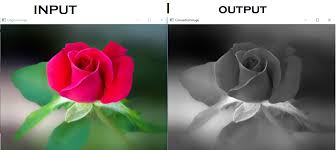
When red/green/blue values are equal .. shade of gray Average combines red/green/blue into one number The average measures how bright the pixel is 0..255. example code below
image = new SimpleImage("flowers.jpg");
for (pixel: image) {
avg = (pixel.getRed() + pixel.getGreen() + pixel.getBlue())/3;
pixel.setRed(avg);
pixel.setGreen(avg);
pixel.setBlue(avg);
}
print(image);
Image Logic and Bluescreen effects
Image Logic: in this section, we learned about if statement, The if/else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed.
having understanding the if statement, we then use it to manipulate images in a very interesting way. one example is converting a specified part of an image from Red to green, thereby leaving other part of image.
Bluescreen effect is a way of substituting pixels of specific location(x,y) in an image with another image. for example, when we change picture’s background from one color to another.
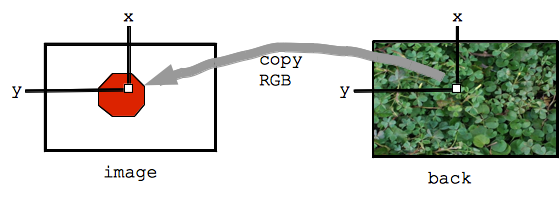
Bluescreen Algorithm
- Say: Two images: image and back
- Detect, say, red pixels in image
- For each red pixel:
- –Consider the pixel at the same x,y in back image
- –Copy over the pixel from back to image
- –What defines how a pixel looks? Three numbers.
- –Copy over the red, green, and blue values
- Result: red areas, substitute in areas from back image
- Adjacent pixels maintained
- example code below….
image = new SimpleImage("stop.jpg");
back = new SimpleImage("leaves.jpg");
for (pixel: image) {
avg = (pixel.getRed() + pixel.getGreen() + pixel.getBlue())/3;
if (pixel.getRed() > avg * 1.5) {
x = pixel.getX();
y = pixel.getY();
pixel2 = back.getPixel(x, y);
pixel.setRed(pixel2.getRed());
pixel.setGreen(pixel2.getGreen());
pixel.setBlue(pixel2.getBlue());
}
}
print(image);
Computer Hardware
Computer hardware is the physical part of the computer e.g CPU and RAM. While Software is a program that enables a computer to perform a specific task. An analogy is “if piano is the hardware, the music is the software.
Modern computers use tiny electronic components which can be etched onto the surface of a silicon chip. the most common electronic component is is the transistor, which works as a sort of amplifying valve for a flow of currents.
A chip is fingernail sized silicon, it can contain billions of transistors. In a computer chip, transistors switch between two binary states — 0 and 1. One computer chip can have millions of transistors continually switching, helping complete complex calculations. In a computer chip, the transistors aren’t isolated.
The three major parts that make up a computer – The CPU, RAM and Persistent Storage. Also these three components are found in laptop, smartphone or tablets.
A CPU – Central processing unit is the brain of the computer, it performs most of the processing inside the computer. The CPU is the active (say: running of code, manipulation of data and so on) part of the computer while other components are the passive part.
Computer stores information as Bytes, a space that data takes up in the computer is measured by byte. One byte is capable of holding a single “a” letter. Both RAM and persistent also stores information as byte in computer.
- 1MB – 1 Million bytes, 1GB – 1 Billion bytes and so on.
RAM – Random Access Memory is a temporary working storage bytes. RAM allows computer to perform many of its everyday tasks, such as loading applications, browsing the internet, editing a spreadsheet, or experiencing the latest game. It is volatile i.e not persistent – gone when power goes off.
Persistent Storage: A long term storage for bytes. It is non volatile storage system i.e bytes that are stored in it don’t get lost when the power goes off. A laptop might use a spinning hard disk for persistent storage of files or It could use a Flash drive also know as a Solid State Disk (SSD), to store bytes on flash chips .
Bit and Bytes
At the smallest scale in the computer, information is stored as bit and bytes.
A bit is the smallest amount of storage you can have on a computer, it just stores 0 and 1, since bits are too small for a computer to store something, then we have a group of 8 bits which is called Bytes. 1 bytes can store one letter e.g. ‘b’, or ‘a’.
- A bit can thus have 2 pattern, in case of ( 0 or 1)
- 2 bits – 4 patterns – (0 0, 0 1, 0 1, 1 1)
- 3 bits = 8 patterns
- 4 bits – 16 patterns
- 5 bits – 32 patterns
- 6 bits – 64 patterns,
- 7 bits – 128 patterns
- 8 bits – 256 patterns
- Mathematically: n bits yields 2n patterns ( 2 to the nth power)
A Bytes
Bytes is just a group of 8 bits, which means with 1 bytes we can have 256 patterns. If we are to count this pattern and relate it to numbers in human pattern, then we have 0, 1, 2…254, 255. The reason why we are not counting to 256 is because 0 already has 1 pattern. that is why when we represent color in code the maximum value is set to 255. Each RGB value are stored in 1 byte in computer
A Kilobytes (KB) is about 1 thousand bytes. for example a small email text is about 2KB. A Megabytes is about 1 Million bytes, an example is MP3 audio. A Gigabyte (GB) is about a billion bytes while A Terabytes (TB) is about a trillion bytes.
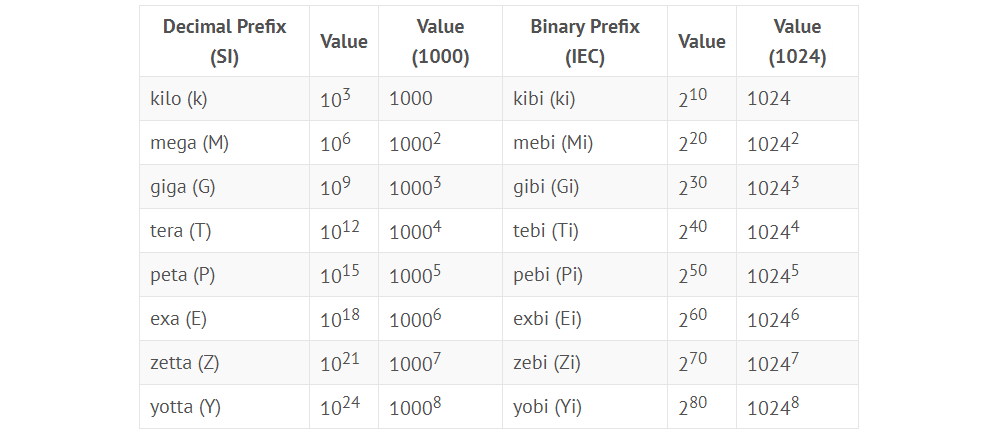
Note: There is also Kibibyte, Mebibyte, Gibibyte and Tebibyte form of representing bytes, you can check this post on Medium for clarity.
A Gigahertz (GHz) is not a measure of bytes, it is a measure of speed, 1 Gigahertz refers to one billion cycles per second and so gigahertz usually come out when talking about how many cycles per second a CPU can do. So processors with higher GHz ratings can theoretically do more in a given unit of time than processors with lower GHz ratings.
Computer Software
Software is the codes that runs on the hardware, it is a set of instruction that tell the computer what to do. Software are generally being run to solve a particular problem. A CPU understands a low level language that is called Machine code, which is the language that is hardwired to the computer hardware.
Machine code is a computer program written in machine language. It uses the instruction set of a particular computer architecture. It is usually written in binary. Machine code is the lowest level of software. Other programming languages are translated into machine code so the computer can execute them. A program is made up of a sequence of millions of these very simple machine code.
The CPU runs instructions using a “fetch-execute” cycle: the CPU gets the first instruction in the sequence, executes it (adding two numbers or whatever), then fetches the next instruction and executes it, and so on.
When the user double click a program file to run it , essentially the block of bytes of the instructions for the program are copied into RAM, and then the CPU is directed to begin running at the first instruction in that area of RAM.
The Operating System of a computer is like a first, supervisory program that begins running when the computer first starts (boot up) . It helps in management, starting and ending other programs. Modern computers can run multiple programs at the same time, Operating system keeps each program run isolated and set RAM for individual task to be carried out.
Computer language
A programmer have to write a code for the computer to perform one or two task. This language used to write and communicate with the computer is called Source code. While source code can’t be understand directly by the computer, it needs to be translated to machine code so that CPU can executes it necessary functions.
We have the Compiled language and Interpreted language.
Compiled language
In this form of language, program is being run from compiled source code. When the programmer writes the source code, he then takes an additional step to convert the source code to a machine code by compiling it first. Compiler looks at the source code and translates the source code into machine code instructions. some example of language that fall in this category is C and C++, they are relatively low level language.
an example of a program written in C++ is Firefox. firefox source code (C++) is converted to machine code by compiler. compiler takes the source code, produces Firefox.exe. The Compilation can be done once and long before the program is run. The end user can’t see the source code as they are distributed in program.exe file in working form. having compiled to program.exe, you cannot recover the source code.
So this means to add features or fix a bug, ideally you want the source code, add a feature in the source code, then run the compiler again to make a new version of the .exe.
Dynamic (Interpreted) language
An interpreted language is a type of programming language for which most of its implementations execute instructions directly and freely, without previously compiling a program into machine-language instructions. The interpreter proceeds through the code given to it, line by line. For each line, the interpreter deconstructs what the line says and perform those actions, piece by piece. some example of interpreted language are Python and Javascript.
Computer Networking
Computer Network is like a phone system where any computer can place a data call to any other computer. A computer can call another computer on the internet to get or send little information.
One way in which a computer cover network is by LAN (Local Area Network), a small scale network, that maybe used to cover a floor of building or a house of 2-50 computers. Two very common examples of LAN technology are Ethernet (which is wired) and Wi-Fi (wireless radio sort of analog to Ethernet)
Ethernet
Ethernet, a very ubiquitous wired LAN, it use wire that is as thick as a drinking straw that terminate in an RJ-45 jack, they are blue or yellow color. Ethernet cable length is limited to 100 meters (keeping it to be locally oriented)
Ethernet send data in form of packets, where it break the large data into packets. The sender reads through all the bits, and sends a pattern of voltage and the receiver reconstructs all the bites to get the packet back. Checksum is a techniques in which error is being detected and corrected, the process involves some little calculations that makes sure each party (sender and receiver of packets) are sum to the same value.
Ethernet share data to many computers by considering each computer’s address. Senders wait for period of silence on the wire, then spreads out on wire, reaching all computers. All computer listen to the wire all the time, pick out packets addressed to them, ignore other packets.
- Ethernet is shared, distrubuted and collaboratives
- Ethernet is insecure since it is shared, another person can listen to the data being sent. To get it secured you can optimize the use of encryption layer of security, again since it is shared the performance can be slow as they are more computer connected to it.
Wi-Fi
Wi-Fi uses same strategy with Ethernet, the hallmark of Ethernet is that you had this shared wire, and only one person really transmit at a time and you want to collectively share it. Wi-fi is the same. In this case instead of wire it has a radio channel in which the air is the medium.
The Internet – TCP/IP
The World Wide Internet is based actually on some Government-funded research in the 70s from the United States, creating some standards called TCP/IP and they are free and open standard. The way Internet works is that every computer has an IP address, Ip address is 4 bytes ( i.e 123.234.235.12 each numbers range from 0 – 255).
Domain names are shorthand for IP addresses. people tends to remember name rather than numbers. for example…say “collinsnote.com” is kind of a shorthand for IP address “123.234.235.12 (huh…just example).
Packet is being transferred by Router, a router us a bit of networking equipment that is connected to two or more networks and it basically shuffles packets back and forth.
Basic of Tables and Spreadsheet
Table are common ways to organize data on the computer, table is being organize into fields, data is stored in rows. You can think of email inbox is being stored in a table on the computer, so the fields in the mail might be, from and to, date, subject and other few things. A CSV refers to a Comma Seperated Values, it’s a standard for storing table data in a text file.
We can have use “&&” to represent and in code, “||” to represent or in code and “!” to represent not in code. Having this features can make us join two or more fields in table and so some more features.
Basic of Spreadsheet
Spreadsheet or worksheet is a file made of rows and columns that help sort, organize, and arrange data efficiently, and calculate numerical data. What makes a spreadsheet software program unique is its ability to calculate values using mathematical formulas and the data in cells. An example of how a spreadsheet may be utilized is creating an overview of your bank’s balance.
Spreadsheet are fantastic invention that make it really easy to do basic kinds of analysis and mathematics.
Computer Security
Computer is like a castle with walls, inside and outside are very different. Bad guys can just access files (bytes) stored inside the computer. typically the bad guys are not crafting some attack just for you, they send in the millions rather crude attacks, just snaring the most clumsy victims. if you avoid most common errors, you will probably be fine.
list of some popular computers attack
- Password Attack
- Phishing attack
- malware attack
Password attack
A password attack is exactly what it sounds like: a third party trying to gain access to your systems by cracking a user’s password.
The attacker could try to guess password on a site, trying to log in again and again all the words in the dictionary.
Therefore avoid having an obvious or commonly used password in the dictionary, a pun or something that someone else might also use.
- Don’t use a bad password for an important site e.g bank
- Don’t re-use passwords across important sites
Phishing Attacks
Phishing is the fraudulent attempt to obtain sensitive information or data, such as usernames, passwords and credit card details, by disguising oneself as a trustworthy entity in an electronic communication. An example of phishing…. is a website trying to imitates the real eBay site or putting a link in an external website and luring one to put his/her password.
- Don’t trust urls in email or random sites, especially when leading to a login page, scrutinize the url as shown in your browser.
- Check for https in the url of the site you are visiting.
Malware Attack
Malware is the collective name for a number of malicious software variants, including viruses, ransomware and spyware. An example…. suppose a bad guy or someone you know send a Plain.TXT or (.JPG) file. The bad guy can write a program and send it to you and get to you to run it on your computer without your consents.
one way to safe from this attack is to be very wary of downloading and running File or program (google the name or source)
Vulnerability is a weakness which can be exploited by a threat actor, such as attacker, to cross privileged boundaries (i.e perform unauthorized actions) with in a computer system.
- keep internet-facing software on auto-update to stay updated
Conclusion
While this post is just a brief overview of what I learned in CS101, I will advise you to consider taking the Course from EDX, so that you have access to vast Lecture and Course resources.
Thanks for reading…..I would appreciate if you can drop a comment in this post.
Super interesante. No sabia nada del curso. Llegué a este blog porque necesitaba algo de ayuda para resolver el ejercicio de speller de CS50. Me gustó mucho.
Thanks, I’m Glad you find it interesting.
have you been able to solve the Speller problem set?
Amazing info! Thank you so much for sharing all this!